As a follow-up to my previous post on Factory vs Constructor Functions in JavaScript, below is a collection of useful examples that aim to illustrate Objects and their related functionalities.
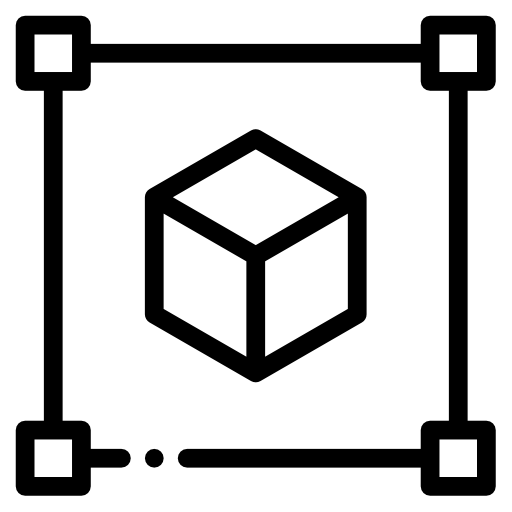
// Obj literal syntax const person = { name: 'Behnam', age: 37 }; // Constructor function function Person(name, age) { this.name = name; this.age = age; } const behnam = new Person('Behnam', 37); // Class syntax (ES6+) class Animal { constructor(name) { this.name = name; } } const bear = new Animal('Bumzy');
Accessing Object Properties
// Dot notation console.log(person.name); // Behnam // Bracket notation console.log(person['age']); // 37
Modifying Object Properties
person.age = 30; // Changing existing property person.city = "Stockholm"; // Adding new property delete person.name; // Deleting a property
Checking if Property Exists
if ('name' in person) { console.log("Name exists!"); }
Looping through Obj properties
for (const key in person) { console.log(`${key}: ${person[key]}`); } // name: Behnam, age: 37 // Object.keys (ES5+) Object.keys(person).forEach((key) => { console.log(`${key}: ${person[key]}`) }); // name: Behnam, age: 37
Object Methods
const calculator = { add: function(a, b) { return a + b; }, subtract(a, b) { return a - b; } }; console.log(calculator.add(5, 3)); // 8 console.log(cakcykatir.subtract(7, 2)); // 5
Object Serialization
The snippet below first converts the person object into a JSON string using JSON.stringify()
. It then parses the JSON string back into a JavaScript object using JSON.parse()
. This helps transferring data between different systems.
const json = JSON.stringify(person); console.log(json); // {"age":37,"name": Behnam} const obj = JSON.parse(json); console.log(obj.age); // 37
Inheritance
We’ll create a basic inheritance example involving Person
and Student
objects.
// Parent object constructor function Person(name, age) { this.name = name; this.age = age; } // Adding a shared method to the Person prototype Person.prototype.sayHello = function () { console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`); }; // Child object constructor inheriting from Person function Student(name, age, grade) { // Call the Person constructor to set name and age Person.call(this, name, age); this.grade = grade; } // Set up the prototype chain for inheritance Student.prototype = Object.create(Person.prototype); Student.prototype.constructor = Student; // Add a unique method to the Student prototype Student.prototype.study = function () { console.log(`${this.name} is studying in grade ${this.grade}.`); }; // Create instances of Person and Student const person = new Person('Alice', 30); const student = new Student('Bob', 18, 12); // Use the inherited and unique methods person.sayHello(); student.sayHello(); student.study();
In this example:
- We have a
Person
constructor function that defines two properties (name
andage
) and a shared methodsayHello()
. - We then create a
Student
constructor function that inherits fromPerson
. It callsPerson.call(this, name, age)
to set the shared properties and usesObject.create(Person.prototype)
to set up the prototype chain for inheritance. - The
Student
constructor adds its unique methodstudy()
to its prototype. - We create instances of both
Person
andStudent
, and then call their methods to demonstrate inheritance. TheStudent
object inherits thesayHello()
method from thePerson
prototype and has its ownstudy()
method.