Lazy loading is a technique employed to enhance website performance by deferring the loading of images until they are necessary and in viewport. In other words, rather than loading all images simultaneously, lazy loading postpones their loading until they are about to be displayed in the visible area of the webpage or when the user scrolls to them. This technique decreases the initial load time of the page and reduces data consumption, leading to quicker and more effective browsing experiences.
In practice, implementing lazy loading for images is a straightforward process accomplished by adding a solitary attribute to your image tag. By setting the loading attribute to “lazy
,” you activate the lazy loading functionality for the image. The browser will then autonomously decide the appropriate timing to download the image, considering its proximity to the visible area of the screen. The primary drawback of this simple lazy loading technique is that the user will encounter an empty space in place of the image until it finishes downloading.
<img src="image.jpg" loading="lazy" />
An Enhanced approach
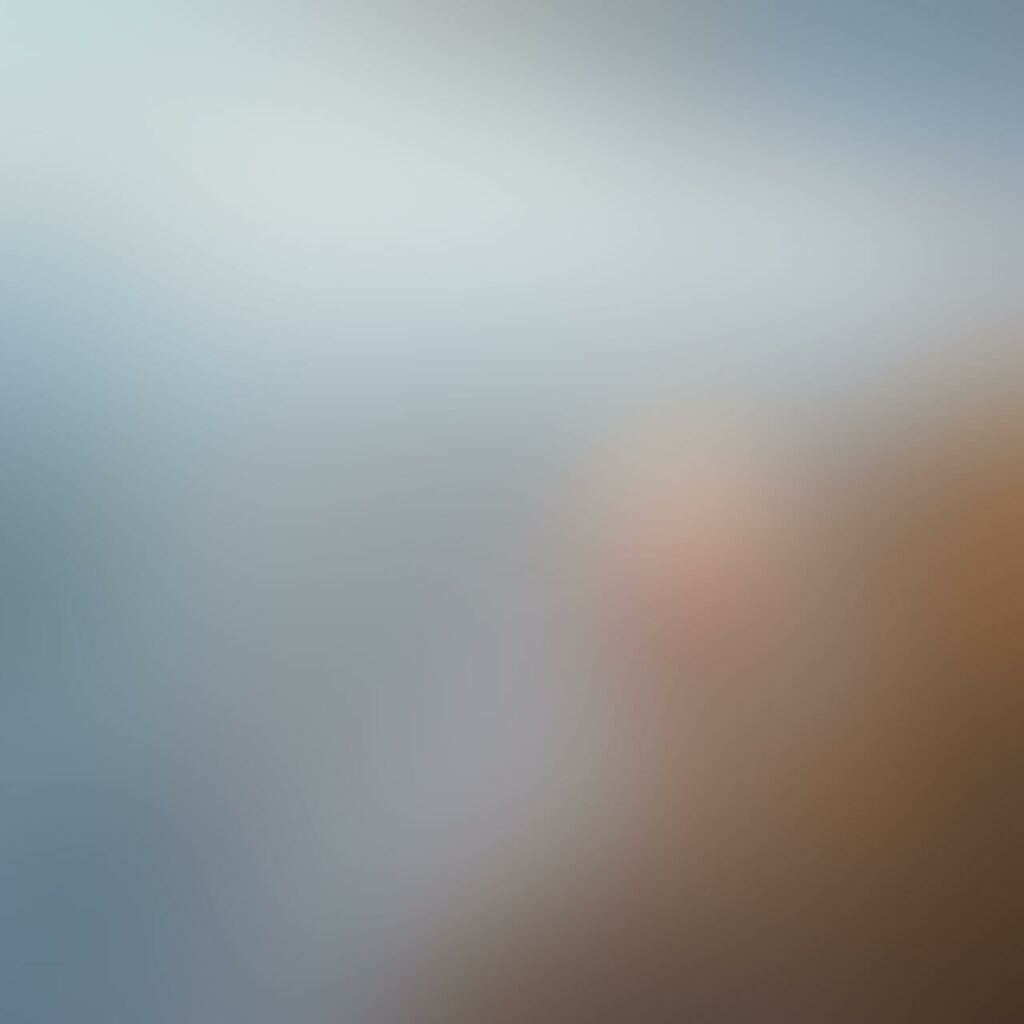
To implement advanced lazy loading, we can generate a small, blurry placeholder image using a tool like ffmpeg
. By setting this placeholder image as the background of a <div>
element, we create a visual placeholder for the full image. To ensure a smooth transition, we can hide the actual <img>
element by default within the <div>
.
To create a placeholder image using ffmpeg, run the following command in the command line:
ffmpeg -i testImg.jpg -vf scale=30:-1 testImg-sm.jpg
This generates a small image that is 30 pixels wide, while maintaining the aspect ratio. The next step is to create the HTML structure with the blurred image as the background of the <div>
, and the full image hidden within it:
<div class="blurred-img"> <img src="testImg.jpg" loading="lazy" /> </div>
To enhance the effect, you can add a CSS filter property to the <div>
to increase the blur:
.blurred-img { filter: blur(10px); }
What is more, you can add a pulsing effect to the placeholder image to indicate loading:
.blurred-img::before { content: ""; position: absolute; inset: 0; opacity: 0; background-color: white; animation: pulse 3.2s infinite; } @keyframes pulse { 0% { opacity: 0; } 50% { opacity: 0.1; } 100% { opacity: 0; } }
To fade in the full image once it is loaded, you can add JavaScript code that listens for the image load event and applies a “loaded” class to the <div>
:
const blurredImageDiv = document.querySelector(".blurred-img") const img = blurredImageDiv.querySelector("img") function loaded() { blurredImageDiv.classList.add("loaded") } if (img.complete) { loaded() } else { img.addEventListener("load", loaded) }
Finally, update the CSS to include transitions and reveal the full image when the “loaded” class is added:
.blurred-img.loaded::before { animation: none; content: none; } .blurred-img img { opacity: 0; transition: opacity 250ms ease-in-out; } .blurred-img.loaded img { opacity: 1; }
With these implementations, the webpage will display a small, blurred placeholder image until the full image is loaded. The full image will then fade in smoothly, enhancing the user experience.